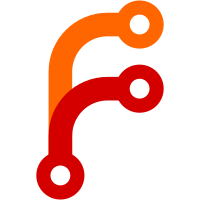
* [ISSUE 1046] UI allows to submit message with empty key & value (#1264)
* [ISSUE 1046] UI allows to submit message with empty key & value
* Update Contract
(cherry picked from commit 4b730eb288
)
* Backend fix
* Refactoring
* Fix nullable & checkstyle
* Fix jsonnullable get
* Remove unnecessary check and add a test
Co-authored-by: Oleg Shur <workshur@gmail.com>
57 lines
1.3 KiB
TypeScript
57 lines
1.3 KiB
TypeScript
import { TopicMessageSchema } from 'generated-sources';
|
|
import Ajv from 'ajv/dist/2020';
|
|
import { upperFirst } from 'lodash';
|
|
|
|
const validateBySchema = (
|
|
value: string,
|
|
schema: string | undefined,
|
|
type: 'key' | 'content'
|
|
) => {
|
|
let errors: string[] = [];
|
|
|
|
if (!value || !schema) {
|
|
return errors;
|
|
}
|
|
|
|
let parcedSchema;
|
|
let parsedValue;
|
|
|
|
try {
|
|
parcedSchema = JSON.parse(schema);
|
|
} catch (e) {
|
|
return [`Error in parsing the "${type}" field schema`];
|
|
}
|
|
if (parcedSchema.type === 'string') {
|
|
return [];
|
|
}
|
|
try {
|
|
parsedValue = JSON.parse(value);
|
|
} catch (e) {
|
|
return [`Error in parsing the "${type}" field value`];
|
|
}
|
|
try {
|
|
const validate = new Ajv().compile(parcedSchema);
|
|
validate(parsedValue);
|
|
if (validate.errors) {
|
|
errors = validate.errors.map(
|
|
({ schemaPath, message }) =>
|
|
`${schemaPath.replace('#', upperFirst(type))} - ${message}`
|
|
);
|
|
}
|
|
} catch (e) {
|
|
return [`${upperFirst(type)} ${e.message}`];
|
|
}
|
|
|
|
return errors;
|
|
};
|
|
|
|
const validateMessage = (
|
|
key: string,
|
|
content: string,
|
|
messageSchema: TopicMessageSchema | undefined
|
|
): string[] => [
|
|
...validateBySchema(key, messageSchema?.key?.schema, 'key'),
|
|
...validateBySchema(content, messageSchema?.value?.schema, 'content'),
|
|
];
|
|
|
|
export default validateMessage;
|