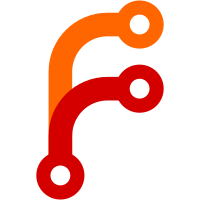
# Conflicts: # kafka-ui-react-app/package-lock.json # kafka-ui-react-app/src/components/Brokers/Brokers.tsx # kafka-ui-react-app/src/components/Brokers/BrokersContainer.ts # kafka-ui-react-app/src/components/Dashboard/ClustersWidget/ClusterWidget.tsx # kafka-ui-react-app/src/components/Dashboard/ClustersWidget/ClustersWidget.tsx # kafka-ui-react-app/src/components/Topics/Details/DetailsContainer.ts # kafka-ui-react-app/src/components/Topics/Details/Messages/Messages.tsx # kafka-ui-react-app/src/components/Topics/List/ListItem.tsx # kafka-ui-react-app/src/components/Topics/New/NewContainer.ts # kafka-ui-react-app/src/components/Topics/TopicsContainer.ts # kafka-ui-react-app/src/redux/actions/actions.ts # kafka-ui-react-app/src/redux/api/clusters.ts # kafka-ui-react-app/src/redux/api/consumerGroups.ts # kafka-ui-react-app/src/redux/api/topics.ts # kafka-ui-react-app/src/redux/interfaces/broker.ts # kafka-ui-react-app/src/redux/interfaces/topic.ts # kafka-ui-react-app/src/redux/reducers/clusters/selectors.ts # kafka-ui-react-app/src/redux/reducers/topics/reducer.ts
49 lines
1.2 KiB
TypeScript
49 lines
1.2 KiB
TypeScript
import React from 'react';
|
|
import cx from 'classnames';
|
|
import { NavLink } from 'react-router-dom';
|
|
import { TopicWithDetailedInfo } from 'redux/interfaces';
|
|
|
|
interface ListItemProps {
|
|
topic: TopicWithDetailedInfo;
|
|
}
|
|
|
|
const ListItem: React.FC<ListItemProps> = ({
|
|
topic: { name, internal, partitions },
|
|
}) => {
|
|
const outOfSyncReplicas = React.useMemo(() => {
|
|
if (partitions === undefined || partitions.length === 0) {
|
|
return 0;
|
|
}
|
|
|
|
return partitions.reduce((memo: number, { replicas }) => {
|
|
const outOfSync = replicas?.filter(({ inSync }) => !inSync);
|
|
return memo + (outOfSync?.length || 0);
|
|
}, 0);
|
|
}, [partitions]);
|
|
|
|
return (
|
|
<tr>
|
|
<td>
|
|
<NavLink
|
|
exact
|
|
to={`topics/${name}`}
|
|
activeClassName="is-active"
|
|
className="title is-6"
|
|
>
|
|
{name}
|
|
</NavLink>
|
|
</td>
|
|
<td>{partitions?.length}</td>
|
|
<td>{outOfSyncReplicas}</td>
|
|
<td>
|
|
<div
|
|
className={cx('tag is-small', internal ? 'is-light' : 'is-success')}
|
|
>
|
|
{internal ? 'Internal' : 'External'}
|
|
</div>
|
|
</td>
|
|
</tr>
|
|
);
|
|
};
|
|
|
|
export default ListItem;
|