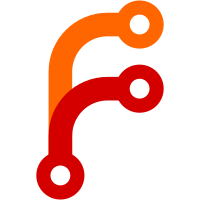
* New hubtest CI for scenarios/parsers from the hub * New `cscli explain` command to visualize parsers/scenarios pipeline Co-authored-by: alteredCoder <kevin@crowdsec.net> Co-authored-by: Sebastien Blot <sebastien@crowdsec.net> Co-authored-by: he2ss <hamza.essahely@gmail.com> Co-authored-by: Cristian Nitescu <cristian@crowdsec.net>
81 lines
1.1 KiB
Go
81 lines
1.1 KiB
Go
package cstest
|
|
|
|
import (
|
|
"fmt"
|
|
"io/ioutil"
|
|
"os"
|
|
)
|
|
|
|
func Copy(sourceFile string, destinationFile string) error {
|
|
input, err := ioutil.ReadFile(sourceFile)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
err = ioutil.WriteFile(destinationFile, input, 0644)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func CopyDir(src string, dest string) error {
|
|
|
|
if dest[:len(src)] == src {
|
|
return fmt.Errorf("Cannot copy a folder into the folder itself!")
|
|
}
|
|
|
|
f, err := os.Open(src)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
file, err := f.Stat()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if !file.IsDir() {
|
|
return fmt.Errorf("Source " + file.Name() + " is not a directory!")
|
|
}
|
|
|
|
err = os.MkdirAll(dest, 0755)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
files, err := ioutil.ReadDir(src)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
for _, f := range files {
|
|
|
|
if f.IsDir() {
|
|
|
|
err = CopyDir(src+"/"+f.Name(), dest+"/"+f.Name())
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
}
|
|
|
|
if !f.IsDir() {
|
|
|
|
content, err := ioutil.ReadFile(src + "/" + f.Name())
|
|
if err != nil {
|
|
return err
|
|
|
|
}
|
|
|
|
err = ioutil.WriteFile(dest+"/"+f.Name(), content, 0755)
|
|
if err != nil {
|
|
return err
|
|
|
|
}
|
|
|
|
}
|
|
|
|
}
|
|
|
|
return nil
|
|
}
|