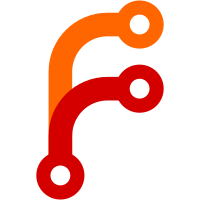
A quality of life update - Mostly. 1.1 - December 21, 2023 - [new] API search for EZTV TV Shows. - [new] config.default.php with default settings. - [new] New option 'imdb_id_search' in 'special' settings in config.php. - [new] New option 'show_zero_seeders' in config.php. - [new] Special result and torrent redirect for IMDb IDs. - [new] Replaced image search with Yahoo! Images. - [new] Styled 'reset' button for search fields. - [tweak] Removed 'raw_output' option. - [tweak] Re-arranged results array to be more logical/easy to use. - [tweak] Re-arranged code for results to do no double checks for search results. - [tweak] Added more user-agents. - [tweak] Torrent results page. - [tweak] Sanitize scraped data earlier in the process. - [tweak] Consistent single quotes for arrays. - [tweak] Consistent spaces, tabs and newlines. - [fix] Inconsistent input height for search field vs search button. - [fix] Better check if a search is currency conversion or not. - [fix] Typos in help.php.
66 lines
2.2 KiB
PHP
66 lines
2.2 KiB
PHP
<?php
|
|
/* ------------------------------------------------------------------------------------
|
|
* Goosle - A meta search engine for private and fast internet fun.
|
|
*
|
|
* COPYRIGHT NOTICE
|
|
* Copyright 2023-2024 Arnan de Gans. All Rights Reserved.
|
|
*
|
|
* COPYRIGHT NOTICES AND ALL THE COMMENTS SHOULD REMAIN INTACT.
|
|
* By using this code you agree to indemnify Arnan de Gans from any
|
|
* liability that might arise from its use.
|
|
------------------------------------------------------------------------------------ */
|
|
class WikipediaRequest extends EngineRequest {
|
|
public function get_request_url() {
|
|
$query_terms = explode(" ", $this->query);
|
|
|
|
// [0] = (wiki|w)
|
|
// [1] = SEARCH TERM
|
|
|
|
unset($query_terms[0]); // Remove first item (w or wiki) from array and encode the rest for Wikipedia
|
|
$this->query = implode(" ", $query_terms);
|
|
|
|
return "https://wikipedia.org/w/api.php?format=json&action=query&prop=extracts%7Cpageimages&exintro&explaintext&redirects=1&pithumbsize=500&titles=".urlencode($this->query);
|
|
}
|
|
|
|
public function parse_results($response) {
|
|
$json_response = json_decode($response, true);
|
|
|
|
if(!empty($json_response)) {
|
|
$result = $json_response['query']['pages'];
|
|
|
|
// Abort on invalid response
|
|
if (!is_array($result)) return array();
|
|
|
|
// Grab first result if there are multiple
|
|
$result = $result[array_key_first($result)];
|
|
|
|
// Page not found
|
|
if (array_key_exists("missing", $result)) {
|
|
return array(
|
|
"title" => "Wiki page not found",
|
|
"text" => "Maybe the page doesn't exist. Try searching on Wikipedia with the link below or search for something else.",
|
|
"source" => "https://wikipedia.org/wiki/Special:Search?go=Go&search=".urlencode($this->query)
|
|
);
|
|
}
|
|
|
|
// Page found
|
|
$response = array(
|
|
"title" => strip_tags(trim($result['title'])),
|
|
"text" => strip_tags(trim($result['extract'])),
|
|
"source" => "https://wikipedia.org/wiki/".urlencode($this->query)
|
|
);
|
|
|
|
if (array_key_exists("thumbnail", $result)) {
|
|
$response['image'] = strip_tags(trim($result['thumbnail']['source']));
|
|
}
|
|
|
|
return $response;
|
|
} else {
|
|
return array(
|
|
"title" => "Sigh...",
|
|
"text" => "Wikipedia could not be loaded. Try again later."
|
|
);
|
|
}
|
|
}
|
|
}
|
|
?>
|